In today’s digital world, people do not just snap photos for memories; they capture hidden data. One of the most incredible pieces of information stored in many images is the geolocation, which includes latitude and longitude. If the device capturing the photo enabled GPS, it can tell us exactly where a photo was taken.
In this post, I will show you how to extract geolocation data from an image using Python. I will specifically work with a photo of a USAID nutrition pack, and after extracting the location, I will plot it on a map. But here is the catch: I will leave it up to you to decide if the pack should be there.
Table of Contents
- How to Extract GPS Coordinates in Python and Plot Them on a Map
- Where Was This Photo Taken?
- Conclusion: The Photo’s True Location
How to Extract GPS Coordinates in Python and Plot Them on a Map
In this section, we will go through the four main steps involved in extracting GPS coordinates from a photo and visualizing it on a map. First, will set up the Python environment with the necessary libraries. Then, we will extract the EXIF data from the image, focus on removing the GPS coordinates, and finally, plot the location on a map.
Step 1: Setting Up Your Python Environment
Before extracting the GPS coordinates, let us prepare your Python environment. We will need a few libraries:
- Pillow: To handle the image file.
- ExifRead: To read the EXIF metadata, including geolocation.
- Folium: To plot the GPS coordinates on an interactive map.
To install these libraries, run the following command:
pip install Pillow ExifRead folium
Code language: Bash (bash)
Now, we are ready to extract information from our photos!
Step 2: Extracting EXIF Data from the Photo
EXIF data is metadata embedded in photos by many cameras and smartphones. It can contain details such as date, camera settings, and GPS coordinates. We can access the latitude and longitude if GPS data is available in the photo.
Here is how you can extract the EXIF data using Python:
import exifread
# Open the image file
with open('nutrition_pack.jpg', 'rb') as f:
tags = exifread.process_file(f)
# Check the tags available
for tag in tags:
print(tag, tags[tag])
Code language: PHP (php)
In the code chunk above, we open the image file 'nutrition_pack.jpg'
in binary mode and use the exifread
library to process its metadata. The process_file()
function extracts the EXIF data, which we then iterate through and print each tag along with its corresponding value. This allows us to see the available metadata in the image, including potential GPS coordinates.
Step 3: Extracting the GPS Coordinates
Now that we have the EXIF data, let us pull out the GPS coordinates. If the photo has geolocation data, it will be in the GPSLatitude
and GPSLongitude
fields. Here is how to extract them:
# Helper function to convert a list of Ratio to float degrees
def dms_to_dd(dms):
degrees = float(dms[0])
minutes = float(dms[1])
seconds = float(dms[2])
return degrees + (minutes / 60.0) + (seconds / 3600.0)
# Updated keys to match your EXIF tag names
lat_key = 'GPS GPSLatitude'
lat_ref_key = 'GPS GPSLatitudeRef'
lon_key = 'GPS GPSLongitude'
lon_ref_key = 'GPS GPSLongitudeRef'
# Check if GPS data exists
if lat_key in tags and lon_key in tags and lat_ref_key in tags and lon_ref_key in tags:
# Extract raw DMS data
lat_values = tags[lat_key].values
lon_values = tags[lon_key].values
# Convert to decimal degrees
latitude = dms_to_dd(lat_values)
longitude = dms_to_dd(lon_values)
# Adjust for hemisphere
if tags[lat_ref_key].printable != 'N':
latitude = -latitude
if tags[lon_ref_key].printable != 'E':
longitude = -longitude
print(f"GPS Coordinates: Latitude = {latitude}, Longitude = {longitude}")
else:
print("No GPS data found!")
Code language: Python (python)
In the code above, we first check whether all four GPS-related tags (GPSLatitude
, GPSLongitude
, and their respective directional references) are present in the image’s EXIF data. If they are, we extract the coordinate values, convert them from degrees–minutes–seconds (DMS) format to decimal degrees, and adjust the signs based on the hemisphere indicators. Finally, the GPS coordinates are printed. If any necessary tags are missing, we print a message stating that no GPS data was found.
Step 4: Plotting the Location on a Map
Now for the fun part! Once we have the GPS coordinates, we plot them on a map. I will use the Folium library to create an interactive map with a marker at the exact location. Here is how to do it:
import folium
# Create a map centered around the coordinates
map_location = folium.Map(location=[latitude, longitude], zoom_start=12)
# Add a marker for the photo location
folium.Marker([latitude, longitude], popup="Photo Location").add_to(map_location)
# Save map to HTML
map_location.save('map_location.html')
Code language: Python (python)
In the code chunk above, we create a map using the folium
library, centered around the extracted GPS coordinates. We then add a marker at the photo’s location and attach a popup labeled “Photo Location.” Finally, the map is saved as an interactive HTML file, allowing us to view it in a web browser and explore the location on the map.
Where Was This Photo Taken?
We have now extracted the geolocation and plotted the coordinates on a map. Here is the question you should ask yourself:
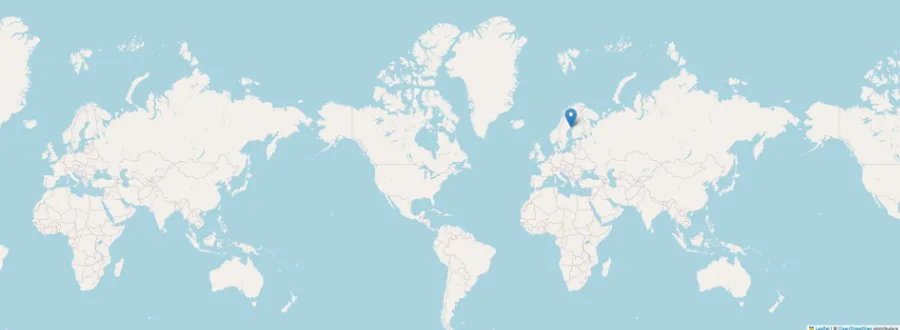
Should the USAID nutrition pack be in this location?
By examining the map and the coordinates, you can make your judgment. Does it make sense for this nutrition pack to be in this specific place? Should it have been placed somewhere else? The photo is of a USAID nutrition pack, and these packs are typically distributed in various places around the world where aid is needed. But is this particular location one that should be receiving this kind of aid?
The coordinates are up to you to interpret, and the map is ready for your eyes to roam. Take a look and think critically: Does this look like a place where this aid should be, or could other places be in more need?
Conclusion: The Photo’s True Location
With just a few lines of Python code, I have extracted hidden geolocation data from a photo, plotted it on an interactive map, and raised the question about aid distribution. Should the USAID nutrition pack be where it was found? After exploring the location on the map, you may have your thoughts about whether this is the right spot for such aid.
Comment below and let me know whether you think the pack should be where it was found. If you believe it should not be there, share this post on social media and help spark the conversation. Also, if you found this post helpful, please share it with others!