When working with large datasets in R, grouping data and counting the number of rows in each group is common. In this post, we will learn how to use data.table count rows by group. In a previous post, we discussed how to get the number of rows in R using data.table. This short tutorial will extend that knowledge by counting rows within groups. First, we will explore how to count rows within a single group using .N
. Second, we will extend this by counting rows across multiple grouping variables to see how data.table handles more complex cases.
Table of Contents
Count Rows by Group in data.table
To count the number of rows by group, we use .N
, which is a built-in feature of data.table. Here is an example:
library(data.table)
# Create a sample data.table
dt <- data.table(category = c("A", "B", "A", "C", "B", "C", "A"), value = 1:7)
# Count rows by group
dt[, .N, by = category]
Code language: PHP (php)
In the code chunk above, we have created a data.table with a categorical column and a numeric column. We then used .N
along with by = category
to count the number of rows for each unique category. The .()
notation is a shorthand for creating a list in data.table. When used inside by
, it allows us to specify multiple grouping variables, ensuring that the row count is calculated for each unique combination of category
and subgroup
.
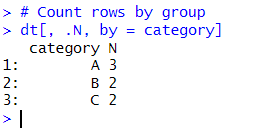
Grouping by Multiple Columns
We can also get the number of rows in data.table by multiple groups:
# Create a new column
dt[, subgroup := c("X", "X", "Y", "Y", "X", "Y", "Y")]
# Count rows by multiple groups
dt[, .N, by = .(category, subgroup)]
Code language: PHP (php)
In the code chunk above, we have added a subgroup
column and then grouped by both category
and subgroup
to count the rows in each combination.
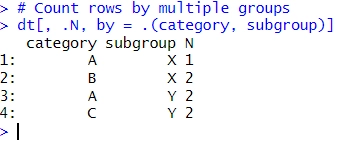
Conclusion
In this post, we learned how to use data.table count rows by group using the handy .N
operator. We also had a look at grouping by multiple columns. This approach is easy to use and well-suited for large datasets. If you found this helpful, feel free to share and comment below!
Resources
Here is some more data.table tutorials that you might find useful:
- How to Filter in data.table in R
- How to Sum Multiple Columns in data.table
- How to Select Columns from data.table in R